This is how I got a cheap wireless PIR driveway monitor to trigger Blue Iris. I’ve been using BI with a Hikvision DS-2CD2032 but had issues with motion detection not working when my car is parked as it mostly masks the path covered by people coming to the front door. When I made it more sensitive it was then more likely to be triggered by birds etc.
So I decided I wanted to use a PIR sensor as its a very mature & cheap technology, and then use a Raspberry Pi as the brains to trigger Blue Iris. In this project I’ve shamelessly borrowed from lots of other people’s projects/blogs/posts and jammed it all together.
I bought a cheap generic Wireless PIR Driveway monitor - Less than £17 from Amazon and available everywhere with various branding but the same overall design,
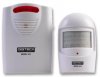
I stuck the PIR sensor so it just covered the door,
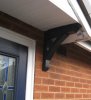
When the PIR is triggered it flashes some LEDs and sounds an alarm on the base unit. I chopped the head off one of the LEDs just leaving 2 pins sticking up, then pushed on 2 jumper leads.
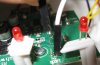
These leads then go to a breadboard with a H11L1 optocoupler which basically uses the voltage from the LEDs being triggered to send a logic signal out to the Raspberry Pi. As its an optocoupler its electrically separated from the Raspberry Pi so I don’t have to worry about zapping the Pi.
The Pi connects to the optocoupler with a 3.3V, ground, and an output which goes into a GPIO pin. Its this pin thats we’re doing all the work with.
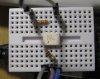
Here’s the complete package,
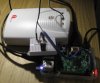
When nothing is triggered the GPIO pin is reading high, and when its triggered its reading low.
I use a small python program which monitors that pin once per second, and if it detects a 0 then logs the result to the screen, and then uses the curl command to call the http trigger address for the camera in Blue Iris. The program then sleeps for 60 seconds because the LEDs flash 4 times in 5 seconds and I don’t want 4 triggers for every event. The code is nothing special but I’ll copy it below - All seems to be working well so far ! Please note the tab indent formatting got stripped when I pasted in the code so you'd need to re-tab it to work, but it should give an idea of what I did.
#!/usr/bin/env python
import time
import subprocess
import RPi.GPIO as GPIO
def trigger_camera():
subprocess.call(['curl','http://192.168.1.82:81/admin?camera=Drive&trigger'])
def log_results(log_det):
local_time = time.asctime(time.localtime(time.time()))
print(local_time,' ',log_det)
GPIO.setmode(GPIO.BCM)
GPIO.setwarnings(False)
GPIO.setup(4, GPIO.IN)
log_results('Started Monitoring')
try:
while True:
#
# Input is 1 by default and 0 when triggered
#
if GPIO.input(4) == 0:
#
# Log the detection
#
log_results('Detected')
#
# Call curl to trigger Blue Iris
#
trigger_camera()
#
# Sleep for 60 seconds after detection to avoid
# repeat triggers for same event
#
time.sleep(60)
#
# Only check the PIR once per second
#
time.sleep(1)
except KeyboardInterrupt:
GPIO.cleanup()
So I decided I wanted to use a PIR sensor as its a very mature & cheap technology, and then use a Raspberry Pi as the brains to trigger Blue Iris. In this project I’ve shamelessly borrowed from lots of other people’s projects/blogs/posts and jammed it all together.
I bought a cheap generic Wireless PIR Driveway monitor - Less than £17 from Amazon and available everywhere with various branding but the same overall design,
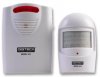
I stuck the PIR sensor so it just covered the door,
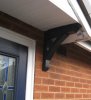
When the PIR is triggered it flashes some LEDs and sounds an alarm on the base unit. I chopped the head off one of the LEDs just leaving 2 pins sticking up, then pushed on 2 jumper leads.
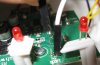
These leads then go to a breadboard with a H11L1 optocoupler which basically uses the voltage from the LEDs being triggered to send a logic signal out to the Raspberry Pi. As its an optocoupler its electrically separated from the Raspberry Pi so I don’t have to worry about zapping the Pi.
The Pi connects to the optocoupler with a 3.3V, ground, and an output which goes into a GPIO pin. Its this pin thats we’re doing all the work with.
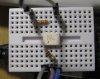
Here’s the complete package,
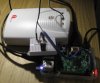
When nothing is triggered the GPIO pin is reading high, and when its triggered its reading low.
I use a small python program which monitors that pin once per second, and if it detects a 0 then logs the result to the screen, and then uses the curl command to call the http trigger address for the camera in Blue Iris. The program then sleeps for 60 seconds because the LEDs flash 4 times in 5 seconds and I don’t want 4 triggers for every event. The code is nothing special but I’ll copy it below - All seems to be working well so far ! Please note the tab indent formatting got stripped when I pasted in the code so you'd need to re-tab it to work, but it should give an idea of what I did.
#!/usr/bin/env python
import time
import subprocess
import RPi.GPIO as GPIO
def trigger_camera():
subprocess.call(['curl','http://192.168.1.82:81/admin?camera=Drive&trigger'])
def log_results(log_det):
local_time = time.asctime(time.localtime(time.time()))
print(local_time,' ',log_det)
GPIO.setmode(GPIO.BCM)
GPIO.setwarnings(False)
GPIO.setup(4, GPIO.IN)
log_results('Started Monitoring')
try:
while True:
#
# Input is 1 by default and 0 when triggered
#
if GPIO.input(4) == 0:
#
# Log the detection
#
log_results('Detected')
#
# Call curl to trigger Blue Iris
#
trigger_camera()
#
# Sleep for 60 seconds after detection to avoid
# repeat triggers for same event
#
time.sleep(60)
#
# Only check the PIR once per second
#
time.sleep(1)
except KeyboardInterrupt:
GPIO.cleanup()